Quickstart#
Now that you installation went ok, let’s start with a small example. Let’s create our first Geodes
object.
[1]:
from pygeodes import Geodes
geodes = Geodes()
Searching for collections#
Then we can start by searching for existing collections, for example with search term sentinel :
[2]:
collections, dataframe = geodes.search_collections("sentinel")
/work/scratch/data/fournih/test_env/lib/python3.11/site-packages/urllib3/connectionpool.py:1099: InsecureRequestWarning: Unverified HTTPS request is being made to host 'geodes-portal.cnes.fr'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings
warnings.warn(
Indexing: 100%|██████████| 111/111 [00:00<00:00, 2032.17it/s]
Let’s see what we found. As a result, we get a collections
object, which is a list of Collection
objects.
[3]:
collections
[3]:
[<Collection id=MUSCATE_Snow_SENTINEL2_L2B-SNOW>,
<Collection id=MUSCATE_SENTINEL2_SENTINEL2_L2A>,
<Collection id=MUSCATE_SENTINEL2_SENTINEL2_L3A>,
<Collection id=MUSCATE_WaterQual_SENTINEL2_L2B-WATER>,
<Collection id=PEPS_S2_L2A>,
<Collection id=PEPS_S2_L1C>,
<Collection id=PEPS_S1_L2>,
<Collection id=PEPS_S1_L1>,
<Collection id=MUSCATE_Snow_MULTISAT_L3B-SNOW>,
<Collection id=TAKE5_SPOT4_L1C>,
<Collection id=MUSCATE_LANDSAT_LANDSAT8_L2A>,
<Collection id=MUSCATE_OSO_RASTER_L3B-OSO>,
<Collection id=TAKE5_SPOT4_L2A>,
<Collection id=MUSCATE_Snow_LANDSAT8_L2B-SNOW>,
<Collection id=PEPS_S3_L1>,
<Collection id=TAKE5_SPOT5_L1C>,
<Collection id=TAKE5_SPOT5_L2A>,
<Collection id=MUSCATE_OSO_VECTOR_L3B-OSO>]
and a dataframe
object, which is a geopandas.GeoDataFrame
.
[4]:
dataframe
[4]:
title | description | collection | |
---|---|---|---|
0 | MUSCATE Snow SENTINEL2 L2B | Theia Snow product is generated from Sentinel-... | <Collection id=MUSCATE_Snow_SENTINEL2_L2B-SNOW> |
1 | MUSCATE SENTINEL2 L2A | The level 2A products correct the data for atm... | <Collection id=MUSCATE_SENTINEL2_SENTINEL2_L2A> |
2 | MUSCATE SENTINEL2 L3A | The products of level 3A provide a monthly syn... | <Collection id=MUSCATE_SENTINEL2_SENTINEL2_L3A> |
3 | MUSCATE WaterQual SENTINEL2 L2B | The processing chain outputs rasters of the co... | <Collection id=MUSCATE_WaterQual_SENTINEL2_L2B... |
4 | PEPS Sentinel-2 L2A tiles | Sentinel-2 L2A tiles acquisition and storage f... | <Collection id=PEPS_S2_L2A> |
5 | PEPS Sentinel-2 L1C tiles | Sentinel-2 L1C tiles acquisition and storage f... | <Collection id=PEPS_S2_L1C> |
6 | PEPS Sentinel-1 Level2 | Sentinel-1 Level-2 consists of geolocated geop... | <Collection id=PEPS_S1_L2> |
7 | PEPS Sentinel-1 Level1 | Sentinel-1 Level-1 products are the baseline p... | <Collection id=PEPS_S1_L1> |
8 | MUSCATE L3B Snow | Level 3 snow products offers annual syntheses ... | <Collection id=MUSCATE_Snow_MULTISAT_L3B-SNOW> |
9 | TAKE5 SPOT4 LEVEL1C | At the end of life of each satellite, CNES iss... | <Collection id=TAKE5_SPOT4_L1C> |
10 | MUSCATE LANDSAT8 L2A | The level 2A products correct the data for atm... | <Collection id=MUSCATE_LANDSAT_LANDSAT8_L2A> |
11 | MUSCATE OSO RASTER | Main characteristics of the OSO Land Cover pro... | <Collection id=MUSCATE_OSO_RASTER_L3B-OSO> |
12 | TAKE5 SPOT4 LEVEL2A | At the end of life of each satellite, CNES iss... | <Collection id=TAKE5_SPOT4_L2A> |
13 | MUSCATE Snow LANDSAT8 L2B | The Theia snow product indicates the snow pres... | <Collection id=MUSCATE_Snow_LANDSAT8_L2B-SNOW> |
14 | GDH Sentinel-3 L1 STM Level-1 products | Sea surface topography measurements to at leas... | <Collection id=PEPS_S3_L1> |
15 | TAKE5 SPOT5 LEVEL1C | At the end of life of each satellite, CNES iss... | <Collection id=TAKE5_SPOT5_L1C> |
16 | TAKE5 SPOT5 LEVEL2A | At the end of life of each satellite, CNES iss... | <Collection id=TAKE5_SPOT5_L2A> |
17 | MUSCATE OSO VECTOR | Main characteristics of the OSO Land Cover pro... | <Collection id=MUSCATE_OSO_VECTOR_L3B-OSO> |
The dataframe let’s see you quickly see what you found, with only a few columns (here description and title), but if you are more comfortable working with raw objects, it’s also possible.
Let’s see how many elements are in these collections.
[5]:
for collection in collections:
print(
f"collection {collection.title} has {collection.summaries.other.get('total_items')} elements"
)
collection MUSCATE Snow SENTINEL2 L2B has 91508 elements
collection MUSCATE SENTINEL2 L2A has 1019637 elements
collection MUSCATE SENTINEL2 L3A has 56011 elements
collection MUSCATE WaterQual SENTINEL2 L2B has 138 elements
collection PEPS Sentinel-2 L2A tiles has 0 elements
collection PEPS Sentinel-2 L1C tiles has 34163668 elements
collection PEPS Sentinel-1 Level2 has 1317955 elements
collection PEPS Sentinel-1 Level1 has 5759206 elements
collection MUSCATE L3B Snow has 667 elements
collection TAKE5 SPOT4 LEVEL1C has 902 elements
collection MUSCATE LANDSAT8 L2A has 27896 elements
collection MUSCATE OSO RASTER has 8 elements
collection TAKE5 SPOT4 LEVEL2A has 814 elements
collection MUSCATE Snow LANDSAT8 L2B has 8708 elements
collection GDH Sentinel-3 L1 STM Level-1 products has 46864 elements
collection TAKE5 SPOT5 LEVEL1C has 3740 elements
collection TAKE5 SPOT5 LEVEL2A has 2953 elements
collection MUSCATE OSO VECTOR has 768 elements
We could want to add columns to our dataframe. To know which columns are available, use collection.list_available_keys()
on a Collection
object :
[6]:
collections[0].list_available_keys()
[6]:
{'assets.snow.description',
'assets.snow.href',
'assets.snow.roles',
'assets.snow.title',
'assets.snow.type',
'assets.wms_capabilities.description',
'assets.wms_capabilities.href',
'assets.wms_capabilities.roles',
'assets.wms_capabilities.title',
'assets.wms_capabilities.type',
'description',
'extent.spatial.bbox',
'extent.temporal.interval',
'id',
'keywords',
'license',
'links',
'providers',
'stac_extensions',
'stac_version',
'summaries.access_url',
'summaries.constellation',
'summaries.contact_email',
'summaries.contact_name',
'summaries.dataset',
'summaries.format',
'summaries.geometry_type',
'summaries.gsd',
'summaries.instruments',
'summaries.item_type',
'summaries.latest',
'summaries.platform',
'summaries.processing:level',
'summaries.temporal_resolution',
'summaries.theme',
'summaries.total_items',
'summaries.variables',
'summaries.version',
'title',
'type'}
Let’s add summaries.total_items
to the dataframe :
[7]:
from pygeodes.utils.formatting import format_collections
new_dataframe = format_collections(
dataframe, columns_to_add={"summaries.total_items"}
)
[8]:
new_dataframe
[8]:
title | description | collection | summaries.total_items | |
---|---|---|---|---|
0 | MUSCATE Snow SENTINEL2 L2B | Theia Snow product is generated from Sentinel-... | <Collection id=MUSCATE_Snow_SENTINEL2_L2B-SNOW> | 91508 |
1 | MUSCATE SENTINEL2 L2A | The level 2A products correct the data for atm... | <Collection id=MUSCATE_SENTINEL2_SENTINEL2_L2A> | 1019637 |
2 | MUSCATE SENTINEL2 L3A | The products of level 3A provide a monthly syn... | <Collection id=MUSCATE_SENTINEL2_SENTINEL2_L3A> | 56011 |
3 | MUSCATE WaterQual SENTINEL2 L2B | The processing chain outputs rasters of the co... | <Collection id=MUSCATE_WaterQual_SENTINEL2_L2B... | 138 |
4 | PEPS Sentinel-2 L2A tiles | Sentinel-2 L2A tiles acquisition and storage f... | <Collection id=PEPS_S2_L2A> | 0 |
5 | PEPS Sentinel-2 L1C tiles | Sentinel-2 L1C tiles acquisition and storage f... | <Collection id=PEPS_S2_L1C> | 34163668 |
6 | PEPS Sentinel-1 Level2 | Sentinel-1 Level-2 consists of geolocated geop... | <Collection id=PEPS_S1_L2> | 1317955 |
7 | PEPS Sentinel-1 Level1 | Sentinel-1 Level-1 products are the baseline p... | <Collection id=PEPS_S1_L1> | 5759206 |
8 | MUSCATE L3B Snow | Level 3 snow products offers annual syntheses ... | <Collection id=MUSCATE_Snow_MULTISAT_L3B-SNOW> | 667 |
9 | TAKE5 SPOT4 LEVEL1C | At the end of life of each satellite, CNES iss... | <Collection id=TAKE5_SPOT4_L1C> | 902 |
10 | MUSCATE LANDSAT8 L2A | The level 2A products correct the data for atm... | <Collection id=MUSCATE_LANDSAT_LANDSAT8_L2A> | 27896 |
11 | MUSCATE OSO RASTER | Main characteristics of the OSO Land Cover pro... | <Collection id=MUSCATE_OSO_RASTER_L3B-OSO> | 8 |
12 | TAKE5 SPOT4 LEVEL2A | At the end of life of each satellite, CNES iss... | <Collection id=TAKE5_SPOT4_L2A> | 814 |
13 | MUSCATE Snow LANDSAT8 L2B | The Theia snow product indicates the snow pres... | <Collection id=MUSCATE_Snow_LANDSAT8_L2B-SNOW> | 8708 |
14 | GDH Sentinel-3 L1 STM Level-1 products | Sea surface topography measurements to at leas... | <Collection id=PEPS_S3_L1> | 46864 |
15 | TAKE5 SPOT5 LEVEL1C | At the end of life of each satellite, CNES iss... | <Collection id=TAKE5_SPOT5_L1C> | 3740 |
16 | TAKE5 SPOT5 LEVEL2A | At the end of life of each satellite, CNES iss... | <Collection id=TAKE5_SPOT5_L2A> | 2953 |
17 | MUSCATE OSO VECTOR | Main characteristics of the OSO Land Cover pro... | <Collection id=MUSCATE_OSO_VECTOR_L3B-OSO> | 768 |
If you wish to produce a fresh new dataframe with your custom columns, use format_collections
on a list of collections :
[9]:
new_dataframe = format_collections(
collections,
columns_to_add={"summaries.constellation", "summaries.instruments"},
)
[10]:
new_dataframe
[10]:
title | summaries.instruments | description | summaries.constellation | collection | |
---|---|---|---|---|---|
0 | MUSCATE Snow SENTINEL2 L2B | [OLI] | Theia Snow product is generated from Sentinel-... | [sentinel-2] | <Collection id=MUSCATE_Snow_SENTINEL2_L2B-SNOW> |
1 | MUSCATE SENTINEL2 L2A | [MSI] | The level 2A products correct the data for atm... | [sentinel-2] | <Collection id=MUSCATE_SENTINEL2_SENTINEL2_L2A> |
2 | MUSCATE SENTINEL2 L3A | [MSI] | The products of level 3A provide a monthly syn... | [sentinel-2] | <Collection id=MUSCATE_SENTINEL2_SENTINEL2_L3A> |
3 | MUSCATE WaterQual SENTINEL2 L2B | [] | The processing chain outputs rasters of the co... | [sentinel-2] | <Collection id=MUSCATE_WaterQual_SENTINEL2_L2B... |
4 | PEPS Sentinel-2 L2A tiles | [MSI] | Sentinel-2 L2A tiles acquisition and storage f... | [sentinel-2] | <Collection id=PEPS_S2_L2A> |
5 | PEPS Sentinel-2 L1C tiles | [MSI] | Sentinel-2 L1C tiles acquisition and storage f... | [sentinel-2] | <Collection id=PEPS_S2_L1C> |
6 | PEPS Sentinel-1 Level2 | [SAR-C] | Sentinel-1 Level-2 consists of geolocated geop... | [sentinel-1] | <Collection id=PEPS_S1_L2> |
7 | PEPS Sentinel-1 Level1 | [SAR-C] | Sentinel-1 Level-1 products are the baseline p... | [sentinel-1] | <Collection id=PEPS_S1_L1> |
8 | MUSCATE L3B Snow | [OLI, MSI] | Level 3 snow products offers annual syntheses ... | [sentinel-2, landsat-8] | <Collection id=MUSCATE_Snow_MULTISAT_L3B-SNOW> |
9 | TAKE5 SPOT4 LEVEL1C | [HRV, HRVIR] | At the end of life of each satellite, CNES iss... | [spot-4] | <Collection id=TAKE5_SPOT4_L1C> |
10 | MUSCATE LANDSAT8 L2A | [OLI] | The level 2A products correct the data for atm... | [landsat-8] | <Collection id=MUSCATE_LANDSAT_LANDSAT8_L2A> |
11 | MUSCATE OSO RASTER | None | Main characteristics of the OSO Land Cover pro... | [sentinel-2] | <Collection id=MUSCATE_OSO_RASTER_L3B-OSO> |
12 | TAKE5 SPOT4 LEVEL2A | [HRV, HRVIR] | At the end of life of each satellite, CNES iss... | [spot-4] | <Collection id=TAKE5_SPOT4_L2A> |
13 | MUSCATE Snow LANDSAT8 L2B | [OLI] | The Theia snow product indicates the snow pres... | [landsat-8] | <Collection id=MUSCATE_Snow_LANDSAT8_L2B-SNOW> |
14 | GDH Sentinel-3 L1 STM Level-1 products | [SRAL] | Sea surface topography measurements to at leas... | [sentinel-3] | <Collection id=PEPS_S3_L1> |
15 | TAKE5 SPOT5 LEVEL1C | [HRG1, HRG2] | At the end of life of each satellite, CNES iss... | [spot-5] | <Collection id=TAKE5_SPOT5_L1C> |
16 | TAKE5 SPOT5 LEVEL2A | [HRG1, HRG2] | At the end of life of each satellite, CNES iss... | [spot-5] | <Collection id=TAKE5_SPOT5_L2A> |
17 | MUSCATE OSO VECTOR | None | Main characteristics of the OSO Land Cover pro... | [sentinel-2] | <Collection id=MUSCATE_OSO_VECTOR_L3B-OSO> |
Note : title
and description
columns are always here by default.
Searching for items#
As for collections, we can search for items. To know which arguments to put in your query, please use :
[11]:
from pygeodes.utils.query import get_requestable_args
print(get_requestable_args())
{'version': 'v8.0', 'attributes': ['dataset (STRING)', 'datetime (DATE_ISO8601)', 'links (STRING)', 'product_validity (BOOLEAN)', 'sci:doi (STRING_ARRAY)', 'no_geometry (BOOLEAN)', 'start_datetime (DATE_ISO8601)', 'end_datetime (DATE_ISO8601)', 'processing:datetime (DATE_ISO8601)', 'processing:lineage (STRING)', 'processing_context (STRING)', 'processing_correction (STRING)', 'processing:version (STRING)', 'bbox (STRING)', 'nb_cols (STRING)', 'nb_rows (STRING)', 'instrument (STRING)', 'platform (STRING)', 'sar:instrument_mode (STRING)', 'processing:level (STRING)', 'sar:polarizations (STRING)', 'sat:orbit_cycle (INTEGER)', 'mission_take_id (INTEGER)', 's2:datatake_id (STRING)', 'sat:relative_orbit (INTEGER)', 'sat:absolute_orbit (LONG)', 'sat:orbit_state (STRING)', 'product:type (STRING)', 'parameter (STRING)', 'pparameter (STRING)', 'product (STRING)', 'temporal_resolution (STRING)', 'classification (STRING)', 'swath (STRING)', 'bands (STRING)', 'grid:code (STRING)', 'eo:cloud_cover (DOUBLE)', 'water_cover (DOUBLE)', 'saturated_defective_pixel (DOUBLE)', 'nodata_pixel (DOUBLE)', 'nb_col_interpolation_error (DOUBLE)', 'ground_useful_pixel (DOUBLE)', 'min_useful_pixel (DOUBLE)', 'sensor_angle (DOUBLE)', 'sensor_pitch (DOUBLE)', 'sensor_roll (DOUBLE)', 'continent_code (STRING_ARRAY)', 'area (DOUBLE)', 'sar:beam_ids (STRING)', 'subTile (STRING)', 'keywords (STRING_ARRAY)', 'political (JSON)']}
We see we can use sat:absolute_orbit
. Let’s search for example those whose orbit direction is 30972:
[12]:
query = {"sat:absolute_orbit": {"eq": 30972}}
items, dataframe = geodes.search_items(query=query, collections=['PEPS_S2_L1C'])
/work/scratch/data/fournih/test_env/lib/python3.11/site-packages/urllib3/connectionpool.py:1099: InsecureRequestWarning: Unverified HTTPS request is being made to host 'geodes-portal.cnes.fr'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings
warnings.warn(
Found 760 items matching your query, returning 80 as get_all parameter is set to False
80 item(s) found for query : {'sat:absolute_orbit': {'eq': 30972}}
Again, we come out with an items
object, and a dataframe
object.
[13]:
items[:10] # printing everything is useless
[13]:
[Item (URN:FEATURE:DATA:gdh:d939b8a4-7f6b-38ba-8925-415a7133a447:V1),
Item (URN:FEATURE:DATA:gdh:8c836d61-26f9-341b-b44d-7e7fa7a74e9b:V1),
Item (URN:FEATURE:DATA:gdh:ea6f4fb7-716a-3d5c-a560-b652e53906a4:V1),
Item (URN:FEATURE:DATA:gdh:8728c52b-c8cc-3237-8cd6-e215836fc505:V1),
Item (URN:FEATURE:DATA:gdh:60d83cde-631a-3d28-b858-251fdb4aae7c:V1),
Item (URN:FEATURE:DATA:gdh:a6d9b9e0-250e-3cad-a77d-5a48608ce653:V1),
Item (URN:FEATURE:DATA:gdh:72989caf-a884-3018-a7ff-c464c835b21b:V1),
Item (URN:FEATURE:DATA:gdh:a449437f-8a51-3a1a-82e8-ea35b046b792:V1),
Item (URN:FEATURE:DATA:gdh:0967199f-0eb1-3189-a19a-fe443e4ad1c9:V1),
Item (URN:FEATURE:DATA:gdh:8550a63f-c516-3411-a5de-10fe155c94b5:V1)]
[14]:
dataframe
[14]:
collection | id | sat:absolute_orbit | item | geometry | |
---|---|---|---|---|---|
0 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:d939b8a4-7f6b-38ba-8925-4... | 30972 | Item (URN:FEATURE:DATA:gdh:d939b8a4-7f6b-38ba-... | POLYGON ((-169.24672 55.84483, -169.22768 56.2... |
1 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:8c836d61-26f9-341b-b44d-7... | 30972 | Item (URN:FEATURE:DATA:gdh:8c836d61-26f9-341b-... | POLYGON ((164.21322 9.86133, 165.08902 9.8624,... |
2 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:ea6f4fb7-716a-3d5c-a560-b... | 30972 | Item (URN:FEATURE:DATA:gdh:ea6f4fb7-716a-3d5c-... | POLYGON ((-101.70029 80.94526, -101.70059 80.9... |
3 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:8728c52b-c8cc-3237-8cd6-e... | 30972 | Item (URN:FEATURE:DATA:gdh:8728c52b-c8cc-3237-... | POLYGON ((-178.52808 54.0505, -178.48457 54.05... |
4 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:60d83cde-631a-3d28-b858-2... | 30972 | Item (URN:FEATURE:DATA:gdh:60d83cde-631a-3d28-... | POLYGON ((-166.97714 63.02793, -164.80704 63.0... |
... | ... | ... | ... | ... | ... |
75 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:5b627fa7-0cb9-3f4b-859f-b... | 30972 | Item (URN:FEATURE:DATA:gdh:5b627fa7-0cb9-3f4b-... | POLYGON ((158.99981 -19.98481, 160.0493 -19.98... |
76 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:ac4f8ba9-73c6-3884-ba9f-c... | 30972 | Item (URN:FEATURE:DATA:gdh:ac4f8ba9-73c6-3884-... | POLYGON ((123.406 -77.56412, 123.37673 -76.580... |
77 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:ebba0242-e75e-339d-8453-6... | 30972 | Item (URN:FEATURE:DATA:gdh:ebba0242-e75e-339d-... | POLYGON ((159.09139 -16.36923, 159.09095 -15.3... |
78 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:932be784-66d0-3c77-8de3-1... | 30972 | Item (URN:FEATURE:DATA:gdh:932be784-66d0-3c77-... | POLYGON ((122.99922 -76.66867, 127.25737 -76.6... |
79 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:f1f066e9-9c4c-389b-9a58-b... | 30972 | Item (URN:FEATURE:DATA:gdh:f1f066e9-9c4c-389b-... | POLYGON ((134.99931 -74.87589, 138.76418 -74.8... |
80 rows × 5 columns
Let’s have a look around our items.
A thing we could want to do is filter them by cloud cover, let’s say between 39 and 40. But this column doesn’t appear in the dataframe. To know which columns are available, use item.list_available_keys()
on an Item
object.
[15]:
items[0].list_available_keys()
[15]:
{'area',
'continent_code',
'dataset',
'datetime',
'end_datetime',
'endpoint_description',
'endpoint_url',
'eo:cloud_cover',
'grid:code',
'id',
'identifier',
'instrument',
'keywords',
'latest',
'physical',
'platform',
'political.continents',
'processing:level',
'processing:version',
'product:timeliness',
'product:type',
'proj:bbox',
'references',
's2:datatake_id',
'sar:instrument_mode',
'sat:absolute_orbit',
'sat:orbit_state',
'sat:relative_orbit',
'sci:doi',
'start_datetime',
'version'}
We see we can use eo:cloud_cover
. We can add it using format_items
.
[16]:
from pygeodes.utils.formatting import format_items
new_dataframe = format_items(
dataframe, columns_to_add={"eo:cloud_cover"}
)
[17]:
new_dataframe
[17]:
collection | id | sat:absolute_orbit | item | geometry | eo:cloud_cover | |
---|---|---|---|---|---|---|
0 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:d939b8a4-7f6b-38ba-8925-4... | 30972 | Item (URN:FEATURE:DATA:gdh:d939b8a4-7f6b-38ba-... | POLYGON ((-169.24672 55.84483, -169.22768 56.2... | 100.000000 |
1 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:8c836d61-26f9-341b-b44d-7... | 30972 | Item (URN:FEATURE:DATA:gdh:8c836d61-26f9-341b-... | POLYGON ((164.21322 9.86133, 165.08902 9.8624,... | 53.467120 |
2 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:ea6f4fb7-716a-3d5c-a560-b... | 30972 | Item (URN:FEATURE:DATA:gdh:ea6f4fb7-716a-3d5c-... | POLYGON ((-101.70029 80.94526, -101.70059 80.9... | 10.542851 |
3 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:8728c52b-c8cc-3237-8cd6-e... | 30972 | Item (URN:FEATURE:DATA:gdh:8728c52b-c8cc-3237-... | POLYGON ((-178.52808 54.0505, -178.48457 54.05... | 100.000000 |
4 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:60d83cde-631a-3d28-b858-2... | 30972 | Item (URN:FEATURE:DATA:gdh:60d83cde-631a-3d28-... | POLYGON ((-166.97714 63.02793, -164.80704 63.0... | 99.999552 |
... | ... | ... | ... | ... | ... | ... |
75 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:5b627fa7-0cb9-3f4b-859f-b... | 30972 | Item (URN:FEATURE:DATA:gdh:5b627fa7-0cb9-3f4b-... | POLYGON ((158.99981 -19.98481, 160.0493 -19.98... | 99.505091 |
76 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:ac4f8ba9-73c6-3884-ba9f-c... | 30972 | Item (URN:FEATURE:DATA:gdh:ac4f8ba9-73c6-3884-... | POLYGON ((123.406 -77.56412, 123.37673 -76.580... | 45.806925 |
77 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:ebba0242-e75e-339d-8453-6... | 30972 | Item (URN:FEATURE:DATA:gdh:ebba0242-e75e-339d-... | POLYGON ((159.09139 -16.36923, 159.09095 -15.3... | 59.269851 |
78 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:932be784-66d0-3c77-8de3-1... | 30972 | Item (URN:FEATURE:DATA:gdh:932be784-66d0-3c77-... | POLYGON ((122.99922 -76.66867, 127.25737 -76.6... | 46.877837 |
79 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:f1f066e9-9c4c-389b-9a58-b... | 30972 | Item (URN:FEATURE:DATA:gdh:f1f066e9-9c4c-389b-... | POLYGON ((134.99931 -74.87589, 138.76418 -74.8... | 18.401654 |
80 rows × 6 columns
We’ve got our new dataframe. Let’s filter :
[18]:
filtered = new_dataframe[
(new_dataframe["eo:cloud_cover"] <= 40)
& (new_dataframe["eo:cloud_cover"] >= 39)
]
[19]:
filtered
[19]:
collection | id | sat:absolute_orbit | item | geometry | eo:cloud_cover | |
---|---|---|---|---|---|---|
73 | PEPS_S2_L1C | URN:FEATURE:DATA:gdh:e4c321cd-3e90-3a97-a357-5... | 30972 | Item (URN:FEATURE:DATA:gdh:e4c321cd-3e90-3a97-... | POLYGON ((170.99981 -15.46502, 171.41679 -15.4... | 39.599036 |
Let’s plot these items :
[20]:
m = filtered.explore()
[21]:
m
[21]:
If you want to have all available columns in your dataframe, just do :
[22]:
full_dataframe = format_items(
items, columns_to_add=items[0].list_available_keys()
)
[23]:
full_dataframe
[23]:
dataset | identifier | collection | references | continent_code | latest | sci:doi | product:timeliness | area | keywords | ... | id | grid:code | proj:bbox | s2:datatake_id | sat:absolute_orbit | end_datetime | version | sat:relative_orbit | item | geometry | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | PEPS_S2_L1C | S2B_MSIL1C_20230209T223659_N0509_R058_T02VNH_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [] | True | [] | Nominal | 980.05545 | [location:northern, season:winter] | ... | URN:FEATURE:DATA:gdh:d939b8a4-7f6b-38ba-8925-4... | T02VNH | -169.24672,55.844830526696,-169.55016,56.31292... | GS2B_20230209T223659_030972_N05.09 | 30972 | 2023-02-09T22:36:59.024Z | 00.00 | 58 | Item (URN:FEATURE:DATA:gdh:d939b8a4-7f6b-38ba-... | POLYGON ((-169.24672 55.84483, -169.22768 56.2... |
1 | PEPS_S2_L1C | S2A_MSIL1C_20210527T233301_N0500_R087_T58PDS_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [] | True | [] | Nominal | 10593.17538 | [location:tropical, location:northern, season:... | ... | URN:FEATURE:DATA:gdh:8c836d61-26f9-341b-b44d-7... | T58PDS | 164.21322248026,9.8613283265692,165.0892981652... | GS2A_20210527T233301_030972_N05.00 | 30972 | 2021-05-27T23:33:01.024Z | 00.00 | 87 | Item (URN:FEATURE:DATA:gdh:8c836d61-26f9-341b-... | POLYGON ((164.21322 9.86133, 165.08902 9.8624,... |
2 | PEPS_S2_L1C | S2A_MSIL1C_20210527T231121_N0500_R087_T13XEL_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [] | True | [] | Nominal | 0.70304 | [location:northern, season:spring] | ... | URN:FEATURE:DATA:gdh:ea6f4fb7-716a-3d5c-a560-b... | T13XEL | -101.70029,80.94526458256,-101.703735,81.05074... | GS2A_20210527T231121_030972_N05.00 | 30972 | 2021-05-27T23:11:21.024Z | 00.00 | 87 | Item (URN:FEATURE:DATA:gdh:ea6f4fb7-716a-3d5c-... | POLYGON ((-101.70029 80.94526, -101.70059 80.9... |
3 | PEPS_S2_L1C | S2A_MSIL1C_20210527T231551_N0500_R087_T01UDA_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [] | True | [] | Nominal | 121.77404 | [location:northern, season:spring] | ... | URN:FEATURE:DATA:gdh:8728c52b-c8cc-3237-8cd6-e... | T01UDA | -178.52808,54.050496442234,-178.40512,54.18715... | GS2A_20210527T231551_030972_N05.00 | 30972 | 2021-05-27T23:15:51.024Z | 00.00 | 87 | Item (URN:FEATURE:DATA:gdh:8728c52b-c8cc-3237-... | POLYGON ((-178.52808 54.0505, -178.48457 54.05... |
4 | PEPS_S2_L1C | S2B_MSIL1C_20230209T223659_N0509_R058_T03VVL_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [] | True | [] | Nominal | 11984.99960 | [location:northern, season:winter] | ... | URN:FEATURE:DATA:gdh:60d83cde-631a-3d28-b858-2... | T03VVL | -166.97714,63.027933225685,-164.80026,64.02701... | GS2B_20230209T223659_030972_N05.09 | 30972 | 2023-02-09T22:36:59.024Z | 00.00 | 58 | Item (URN:FEATURE:DATA:gdh:60d83cde-631a-3d28-... | POLYGON ((-166.97714 63.02793, -164.80704 63.0... |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
75 | PEPS_S2_L1C | S2A_MSIL1C_20210527T233821_N0500_R087_T57KWU_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [] | True | [] | Nominal | 12100.69302 | [location:tropical, location:southern, season:... | ... | URN:FEATURE:DATA:gdh:5b627fa7-0cb9-3f4b-859f-b... | T57KWU | 158.9998088233,-19.984806533577,160.0429308562... | GS2A_20210527T233821_030972_N05.00 | 30972 | 2021-05-27T23:38:21.024Z | 00.00 | 87 | Item (URN:FEATURE:DATA:gdh:5b627fa7-0cb9-3f4b-... | POLYGON ((158.99981 -19.98481, 160.0493 -19.98... |
76 | PEPS_S2_L1C | S2B_MSIL1C_20230209T231349_N0509_R058_T51CVQ_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [AN] | True | [] | Nominal | 6682.91998 | [location:southern, season:summer] | ... | URN:FEATURE:DATA:gdh:ac4f8ba9-73c6-3884-ba9f-c... | T51CVQ | 123.40600037096,-77.564122823385,120.991597763... | GS2B_20230209T231349_030972_N05.09 | 30972 | 2023-02-09T23:13:49.024Z | 00.00 | 58 | Item (URN:FEATURE:DATA:gdh:ac4f8ba9-73c6-3884-... | POLYGON ((123.406 -77.56412, 123.37673 -76.580... |
77 | PEPS_S2_L1C | S2A_MSIL1C_20210527T233821_N0500_R087_T57LVC_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [] | True | [] | Nominal | 6428.01994 | [location:tropical, location:southern, season:... | ... | URN:FEATURE:DATA:gdh:ebba0242-e75e-339d-8453-6... | T57LVC | 159.09139182667,-16.369226028494,158.547456486... | GS2A_20210527T233821_030972_N05.00 | 30972 | 2021-05-27T23:38:21.024Z | 00.00 | 87 | Item (URN:FEATURE:DATA:gdh:ebba0242-e75e-339d-... | POLYGON ((159.09139 -16.36923, 159.09095 -15.3... |
78 | PEPS_S2_L1C | S2B_MSIL1C_20230209T231349_N0509_R058_T51CWR_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [AN] | True | [] | Nominal | 11939.43830 | [location:southern, season:summer] | ... | URN:FEATURE:DATA:gdh:932be784-66d0-3c77-8de3-1... | T51CWR | 122.9992229898,-76.668671848558,126.9712829074... | GS2B_20230209T231349_030972_N05.09 | 30972 | 2023-02-09T23:13:49.024Z | 00.00 | 58 | Item (URN:FEATURE:DATA:gdh:932be784-66d0-3c77-... | POLYGON ((122.99922 -76.66867, 127.25737 -76.6... |
79 | PEPS_S2_L1C | S2B_MSIL1C_20230209T231349_N0509_R058_T53CNT_2... | PEPS_S2_L1C | [{'license': 'Free of Charge', 'author': 'Natu... | [AN] | True | [] | Nominal | 11947.81665 | [location:southern, season:summer] | ... | URN:FEATURE:DATA:gdh:f1f066e9-9c4c-389b-9a58-b... | T53CNT | 134.99931327677,-74.875894734017,138.540447574... | GS2B_20230209T231349_030972_N05.09 | 30972 | 2023-02-09T23:13:49.024Z | 00.00 | 58 | Item (URN:FEATURE:DATA:gdh:f1f066e9-9c4c-389b-... | POLYGON ((134.99931 -74.87589, 138.76418 -74.8... |
80 rows × 34 columns
Providing an api key#
The next parts involve requests that require an api-key. You can register one using the following method. We will also set a default download directory, for later. We use a file config.json
formed as follows :
{"api_key" : "MyApiKey","download_dir" : "/tmp"}
[24]:
from pygeodes import Config
conf = Config.from_file("config.json")
geodes.set_conf(conf)
Other ways to configure pygeodes are described in configuration.
Quicklook#
Now we can have a look at our items.
[25]:
for item in filtered["item"]:
print(f"Quicklook of {item}")
item.show_quicklook()
Quicklook of Item (URN:FEATURE:DATA:gdh:e4c321cd-3e90-3a97-a357-5d64ca3e8e49:V1)
/work/scratch/data/fournih/test_env/lib/python3.11/site-packages/urllib3/connectionpool.py:1099: InsecureRequestWarning: Unverified HTTPS request is being made to host 'geodes-portal.cnes.fr'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/latest/advanced-usage.html#tls-warnings
warnings.warn(
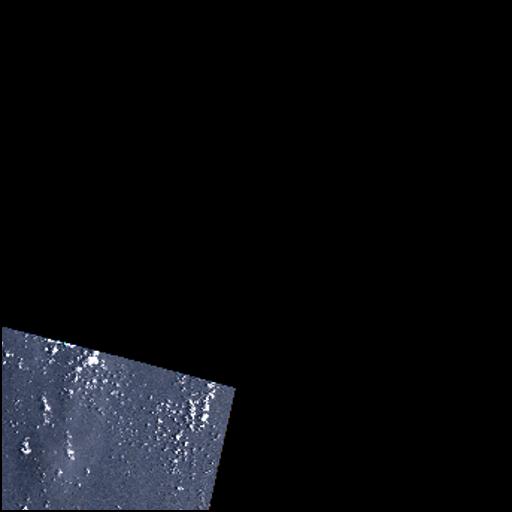
Downloading items#
Now we could want to download these items for further use :
[ ]:
for item in filtered["item"]:
item.download_archive()
As we provided /tmp
as default download dir, the downloads are stored in this folder.